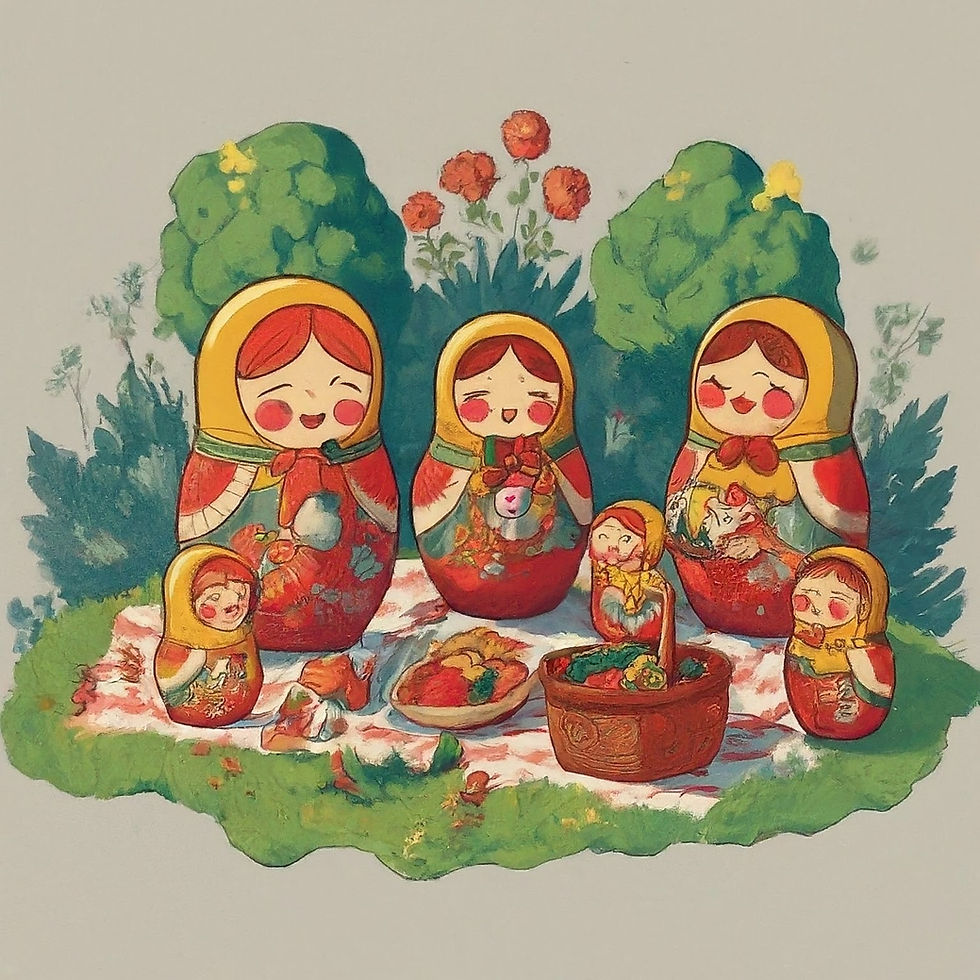
Recursion is a powerful programming concept that involves a function calling itself directly or indirectly this is extremely necessary concept to build recurrent neural networks for machine learning but the fact is that your imagination is the limit.
Analogy: It's like a Russian Matryoshka doll, where each doll contains a smaller version of itself. This nesting of functions or dolls continues until a base case is reached, which is the smallest doll that doesn't contain another doll.
Understanding Recursion
To understand recursion, let's consider the example of calculating a factorial. The factorial of a non-negative integer n is the product of all positive integers less than or equal to n. For example, the factorial of 5 (denoted as 5!) is 5 4 3 2 1 = 120.
A recursive function to calculate the factorial can be defined as follows:
Python language
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
In this code, the factorial function calls itself with n - 1 until n reaches 0. This is the base case, where the function returns 1. The function then multiplies each value of n with the result of the recursive call, eventually calculating the factorial.
The Benefits and Pitfalls of Recursion
Recursion can be a concise and elegant way to solve problems, especially those that can be naturally expressed in a recursive manner. However, it's important to be aware of the potential pitfalls:
Stack Overflow: Recursion can lead to stack overflow errors if the depth of the recursion exceeds the available stack space. This can happen with large input values or inefficient recursive implementations.
Performance Overhead: Recursive function calls can have performance overhead due to the function call mechanism. In some cases, iterative solutions might be more efficient.
When to Use Recursion
Recursion is often used in algorithms that involve dividing a problem into smaller subproblems of the same type. Examples include:
Sorting algorithms: Merge sort and quicksort are recursive algorithms that divide the input array into smaller subarrays.
Tree and graph traversal: Recursive algorithms are commonly used to traverse tree and graph data structures.
Mathematical calculations: Factorials, Fibonacci numbers, and other mathematical functions can be efficiently calculated using recursion.
Conclusion
Recursion is a powerful concept that can be applied to solve a wide range of problems. By understanding the principles of recursion and being aware of its potential drawbacks, you can effectively use it in your programming endeavors.
Here is a basic practical example to help you grasp this concept:
Creating a Simple Countdown App with JavaScript and Velo
Understanding the Requirements
We'll create a simple countdown app where:
The user inputs a starting value.
The countdown starts from that value and decreases by 1 every second.
The countdown stops when it reaches 0.
JavaScript Code
import wixData from 'wix-data';
export function startCountdown(count) {
const countdownElement = $w('#countdown');
let timer = count;
countdownElement.text = timer;
const intervalId = setInterval(() => {
timer--;
countdownElement.text = timer;
if (timer === 0) {
clearInterval(intervalId);
countdownElement.text = "Countdown finished!";
}
}, 1000);
}
Use code with caution.
Velo Interface
Create an input element for the user to enter the starting value.
Create a text element to display the countdown.
Create a button to start the countdown.
Add an onClick event handler to the button that calls the startCountdown function with the value from the input element.
Explanation
startCountdown function: Takes the starting count as input.
Initializes variables: countdownElement for displaying the countdown, timer to store the current count, and intervalId to store the interval ID.
Sets initial countdown value: Displays the initial count in the countdown element.
Starts the interval: Creates an interval that runs every second.
Decrements timer: Decrements the timer by 1 and updates the countdown element.
Checks for finish: If the timer reaches 0, clears the interval and displays "Countdown finished!".
Additional Considerations
Input validation: You might want to add input validation to ensure the user enters a valid number.
User interface: You can enhance the user interface by adding styling, progress bars, or other visual elements.
Reset functionality: Consider adding a button to reset the countdown.
Error handling: Implement error handling for unexpected scenarios.
This basic structure provides a foundation for a simple countdown app. You can expand on it based on your specific requirements and design preferences.