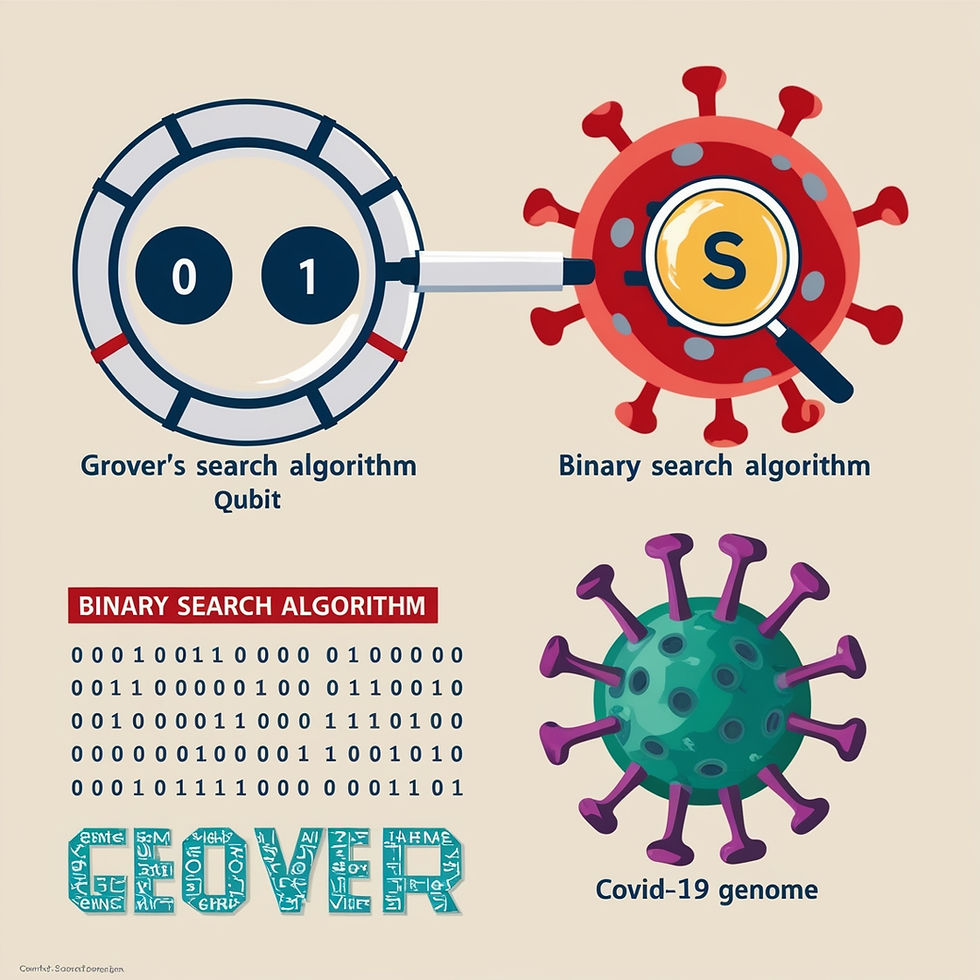
Introduction
We have had so much joy exploring these 2 algorithm and making concrete comparisons between the two, we must share it with you! let's explore them together:
As data continues to grow exponentially, efficient search algorithms have become more critical than ever. For structured data, Binary Search has been the go-to solution for decades due to its efficiency and simplicity. However, with the rise of quantum computing, Grover’s Algorithm promises to revolutionize search tasks, particularly for unstructured data.
In this article, we'll compare Grover's Algorithm, a quantum search algorithm, with Binary Search, a classical algorithm, using a real-world dataset: the complete genome sequence of SARS-CoV-2, the virus responsible for COVID-19. We’ll explore their implementations, use cases, and conclude which one performs better for genomic data.
Dataset: The COVID-19 Genome Sequence
The complete genome sequence of SARS-CoV-2 is about 29,903 base pairs long and is widely used in bioinformatics research. For this comparison, we’ll use the genome data available from the National Center for Biotechnology Information (NCBI).
To begin, we download and parse the genome data.
Step 1: Download the COVID-19 Genome Data using this link or directly in the code with Python:
import requests
# Download the COVID-19 genome data from NCBI
url = "https://api.ncbi.nlm.nih.gov/datasets/v1/genome/accession/NC_045512.2/download"
response = requests.get(url)
# Save the genome sequence to a file
if response.status_code == 200:
with open('sars_cov_2_genome.fasta', 'wb') as f:
f.write(response.content)
print("Genome data successfully downloaded and saved.")
else:
print(f"Failed to download genome data. Status code: {response.status_code}")
Step 2: Parse the Genome Sequence
The genome data is stored in FASTA format. We will now parse it and extract the nucleotide sequence.
# Python
# Function to parse the FASTA file
def parse_fasta(file_path):
with open(file_path, 'r') as f:
lines = f.readlines()
# Remove the header line and join the rest (which contains the genome sequence)
genome_sequence = ''.join([line.strip() for line in lines if not line.startswith('>')])
return genome_sequence
# Parse the SARS-CoV-2 genome sequence
genome_data = parse_fasta('sars_cov_2_genome.fasta')
print(f"Genome Data Length: {len(genome_data)}") # Should print 29,903 base pairs
Implementing the Search Algorithms
Now that we have the genome data, let's implement both search algorithms to search for specific nucleotide sequences (k-mers) in the genome.
A Quantum vs Classic Showdown with COVID-19 Genome Data
Grover’s Algorithm: Quantum Search
Grover’s Algorithm provides a quadratic speedup over classical search algorithms for unstructured data, offering a time complexity of O(N)O(sqrt N)O(N) compared to O(N)O(N)O(N) for a linear search.
Here, we will implement Grover’s Algorithm using Qiskit, IBM’s quantum computing framework. The implementation assumes that the genome data is unstructured, making it a great candidate for a quantum search algorithm.
# Python
from qiskit import QuantumCircuit, Aer, execute
from math import pi
# Grover's Oracle - a simple oracle that checks if an item matches the target
def grovers_oracle(circuit, qr, target_index, n):
for i in range(n):
if target_index[i] == '0':
circuit.x(qr[i])
circuit.h(qr)
circuit.mcx([qr[i] for i in range(n-1)], qr[n-1])
circuit.h(qr)
for i in range(n):
if target_index[i] == '0':
circuit.x(qr[i])
# Grover's Diffusion Operator
def grovers_diffusion(circuit, qr, n):
circuit.h(qr)
circuit.x(qr)
circuit.h(qr[n-1])
circuit.mcx([qr[i] for i in range(n-1)], qr[n-1])
circuit.h(qr[n-1])
circuit.x(qr)
circuit.h(qr)
# Grover's Algorithm
def grovers_algorithm(n, target_index):
qr = QuantumCircuit(n)
# Initialize qubits
qr.h(range(n))
# Apply Oracle and Diffusion
grovers_oracle(qr, range(n), target_index, n)
grovers_diffusion(qr, range(n), n)
qr.measure_all()
simulator = Aer.get_backend('qasm_simulator')
result = execute(qr, simulator, shots=1024).result()
counts = result.get_counts()
print(counts)
# Example search for a k-mer (binary representation of index in genome)
target_index = '101011' # Binary representation of the target index in the genome
grovers_algorithm(6, target_index)
Binary Search: Classical Approach
For Binary Search, we assume the genome data is sorted (although DNA sequences are not naturally sorted, this assumption is made for the algorithm to work efficiently). Binary Search performs efficiently with a time complexity of O(log N)O(log N)O(log N), making it the preferred method for ordered datasets.
We’ll split the genome data into smaller substrings, referred to as k-mers (of length 10 for this example), and search for a specific k-mer.
# Python
# Function to implement binary search
def binary_search(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
# Split genome data into k-mers of length 10
k = 10
kmers = [genome_data[i:i+k] for i in range(len(genome_data) - k + 1)]
kmers.sort() # Sorting the k-mers to apply binary search
# Search for a specific k-mer
target_kmer = 'ATGCATGCAT'
index = binary_search(kmers, target_kmer)
if index != -1:
print(f"K-mer '{target_kmer}' found at index {index}")
else:
print(f"K-mer '{target_kmer}' not found")
Comparison of Grover’s Algorithm vs Binary Search
With both search algorithms implemented, let's summarize their performance and discuss their use cases, this is what we call A Quantum vs Classic Showdown with COVID-19 Genome Data, this will deliver insights on how will quantum computation help to derive large complex patterns in the future.
Why Grover’s Algorithm Is Better for DNA Search
Unstructured Data: DNA sequences, like most real-world data, are not naturally ordered. This makes Binary Search less efficient unless we first sort the data (which is computationally expensive).
Scalability: Grover's Algorithm performs well on unstructured datasets like the genome data, with a time complexity of O(N)O(sqrt N)O(N), which makes it advantageous for large datasets.
Quantum Speedup: As the dataset grows exponentially (which is common in genomic data), Grover's quadratic speedup over classical search algorithms becomes more significant.
However, Grover’s Algorithm is probabilistic, which means it may need to be repeated for high accuracy, unlike Binary Search, which is deterministic.
Algorithm Comparison Table
Algorithm | Time Complexity | Result Accuracy | Best Use Case |
Binary Search | O(logN)O(log N)O(logN) | 100% accuracy | Sorted datasets, structured data |
Grover’s Algorithm | O(N)O(sqrt N)O(N) | Probabilistic | Unstructured data, exponentially large datasets |
Limitations of Grover's Algorithm: It's worth mentioning that Grover's Algorithm is still in its early stages of development. Implementing it on real quantum computers remains challenging due to factors like decoherence.
A short explanation on quantum decoherence:
Quantum decoherence is the process by which a quantum system loses its quantum properties and behaves more like a classical system. In a quantum state, particles can exist in superpositions—simultaneously being in multiple states. However, when a quantum system interacts with its environment (even slightly), these superpositions break down, and the system "decoheres," transitioning into a definite state that aligns with classical physics.
This loss of coherence is critical because it explains why we don't observe quantum phenomena, like superposition or entanglement, in our everyday macroscopic world. Decoherence helps bridge the gap between quantum and classical mechanics, but it doesn’t explain the actual collapse of the wave function, which is still addressed by the measurement problem in quantum mechanics.
If you would like to have a short refresh on quantum principals we have put together a short article on this here: https://www.acebuddy.co/post/quantum-principals-for-starters
Conclusion
Both Grover’s Algorithm and Binary Search have their merits, but their ideal use cases differ. Binary Search is incredibly efficient for structured, sorted datasets, where it guarantees high accuracy. However, when dealing with unstructured data such as DNA sequences, Grover's Algorithm provides a significant quantum speedup.
In the context of genomic searches, Grover's Algorithm offers exciting potential as quantum computing continues to advance. As the size and complexity of genomic datasets grow, the quadratic speedup of Grover's Algorithm will become even more valuable, making it a strong candidate for future DNA sequence searches.